https://leetcode.com/problems/add-two-numbers/
Add Two Numbers - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제
You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order, and each of their nodes contains a single digit. Add the two numbers and return the sum as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
두개의 비어있지 않은, 한자리 양수로 된 연결리스트. 각 수는 역순으로 저장되어있다. 두 수를 더해서 연결리스트 형태로 return하기.
Example 1:
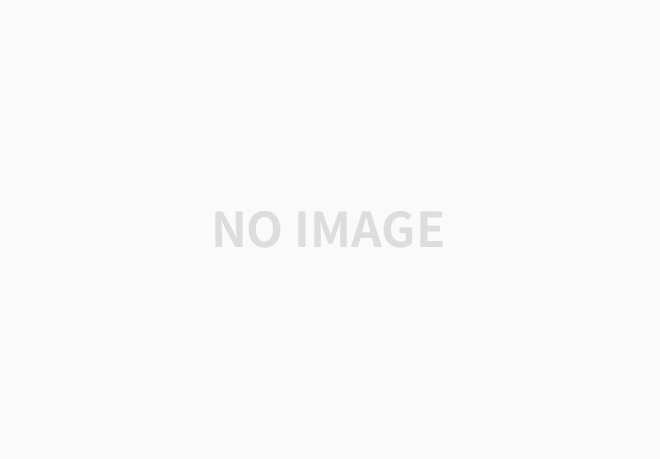
Input: l1 = [2,4,3], l2 = [5,6,4]
Output: [7,0,8]
Explanation: 342 + 465 = 807.
Example 2:
Input: l1 = [0], l2 = [0]
Output: [0]
Example 3:
Input: l1 = [9,9,9,9,9,9,9], l2 = [9,9,9,9]
Output: [8,9,9,9,0,0,0,1]
Constraints:
- The number of nodes in each linked list is in the range [1, 100].
- 0 <= Node.val <= 9
- It is guaranteed that the list represents a number that does not have leading zeros.
/**
* Definition for singly-linked list.
* function ListNode(val, next) {
* this.val = (val===undefined ? 0 : val)
* this.next = (next===undefined ? null : next)
* }
*/
/**
* @param {ListNode} l1
* @param {ListNode} l2
* @return {ListNode}
};
*/
var addTwoNumbers = function(l1, l2) {
};
풀이
연결리스트 문제. 위의 ListNode의 구조를 이용해 해결함.
var addTwoNumbers = function(l1, l2) {
let List = new ListNode(0);
let head = List;
let sum=0;
let carry=0;
//l1가 null이 아니거나, l2가 null이 아니거나, sum이 0보다 크면 반복
while(l1!==null|l2!==null||sum>0){
if(l1!==null){ //l1이 null이 아니면
sum+=l1.val; //sum에 l1의 val을 더하고
l1=l1.next; //다음 노드로 넘어감
}
if(l2!==null){
sum+=l2.val;
l2=l2.next;
}
if(sum>=10){ //sum이 10이상이 되면
carry=1; //carry=1 (한자리수들의 덧셈이므로 1을 초과하진 않음)
sum-=10; //sum에서 10 빼주기
}
head.next=new ListNode(sum); //val이 sum인 리스트를 만들고 head의 next로 넘겨줌
head=head.next; //다음 노드로 이동
sum=carry;
carry=0;
}
return List.next;
};
여기서 val head=List; 를 해주는 이유는 while문 안에서 head를 이용해서 리스트들을 연결해주고, 마지막에 List.next를 리턴하기 위함이다. (List는 현재 맨 앞인 0을 가리키고 있기 때문에 List.next를 리턴해준다.)
'Algorithm > Problem Solving' 카테고리의 다른 글
[Python] 백준/BOJ - 5885번: 거스름돈 (0) | 2021.10.15 |
---|---|
[Python] 백준/BOJ - 11399번: ATM (0) | 2021.10.09 |
[JS] 봉우리 (0) | 2021.09.09 |
[JS] 일곱 난쟁이 (0) | 2021.09.09 |
[JS] LeetCode - 53. Maximum Subarray / 카데인 알고리즘(Kadane's Algorithm) (0) | 2021.09.08 |